vue入门
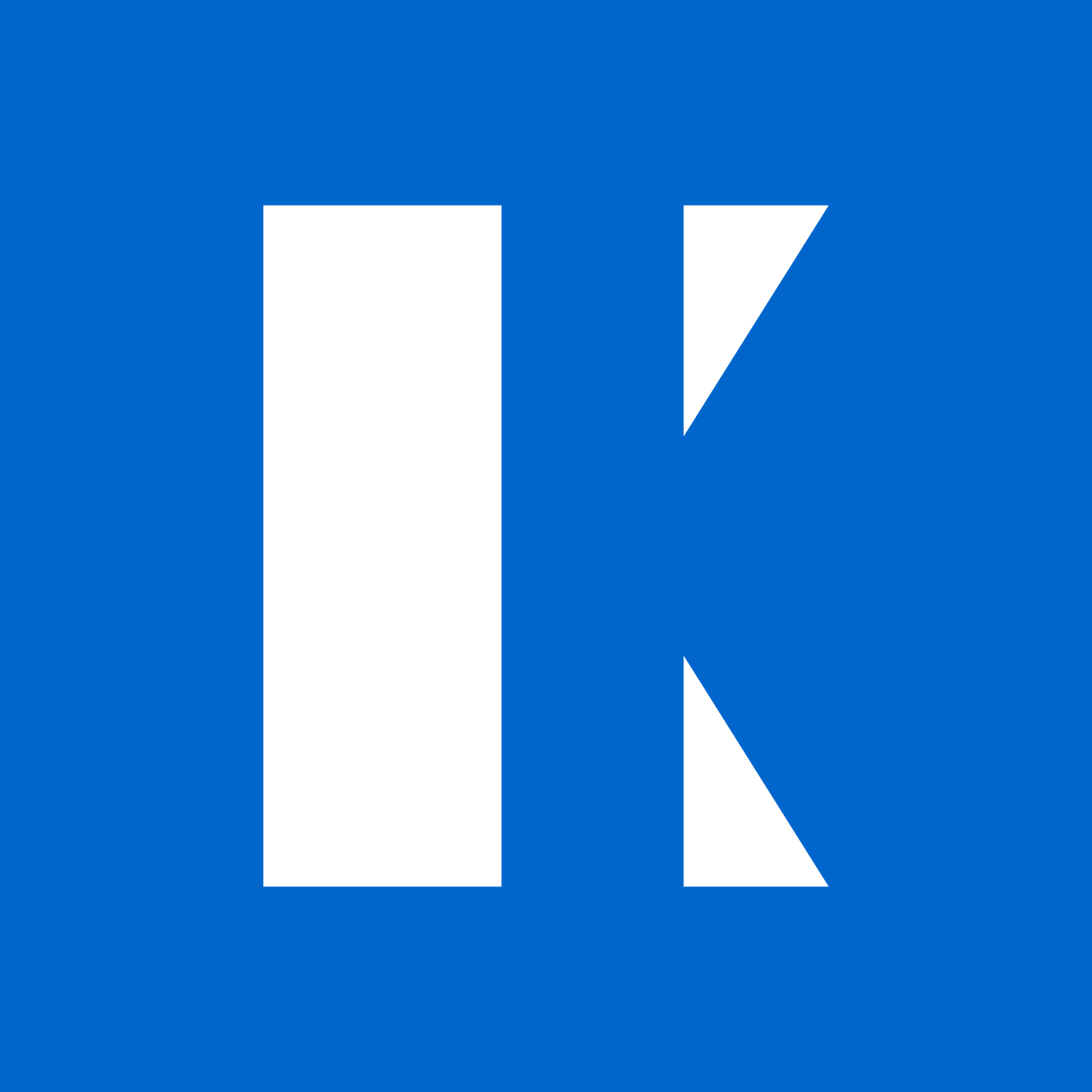
vue入门
一、vue基础
1.1 vue简介
- JavaScript框架
- 简化Dom操作
- 响应式数据驱动
1.2 第一个vue程序
文档传送门:https://cn.vuejs.org/
- 导入开发版本的 Vue.js
- 创建 Vue 实例对象,设置 el 属性和 data 属性
- 使用简洁的模板语法把数据渲染到页面上
1 |
|
1.3 el:挂载点
el 是用来设置 Vue 实例挂载(管理)的元素
Vue 实例的作用范围是什么呢?
Vue 会管理 el 宣子昂命中的元素及其内部的后代元素
是否可以使用其他选择器?
可以使用其他选择器,但是建议使用 ID选择器
是否可以设置其他的 dom 元素呢?
可以使用其他的双标签,不能使用 html 和 body
1 |
|
1.4 data:数据对象
- Vue 中用到的数据定义在 data 中
- data 中可以写复杂类型的数据
- 渲染复杂类型数据时,遵守 js 的语法即可
1 |
|
二、本地应用
2.1 内容绑定,事件绑定
v-text
- v-text 指令的作用时:设置标签的内容(textContent)
- 默认写法会替换全部内容,使用差值表达式可以替换指定内容
- 支持写表达式
1 |
|
v-html
- v-html 指令的作用是:设置元素的 innerHTML
- 内容中有 html 的结构会被解析为标签
- v-html 指令无论内容时什么,指挥解析为文本
- 解析文本使用 v-text,需要解析 html 结构使用 v-html
1 |
|
v-on
- v-on 指令的作用是:为元素绑定事件
- 事件名不需要写 on
- 指令可以简写为 @
- 绑定的方法定义在 methods 属性中
- 方法内部通过 this 关键字可以访问定义在 data 中数据
1 |
|
实例
- 创建 Vue 实例时:el(挂载点),data(数据),methods(方法)
- v-on 指令的作用时绑定事件,简写为 @
- 方法中通过 this ,关键字获取 data 中的数据
- v-text 指令的作用时:设置元素的文本值
- v-html 指令的作用是:设置元素的 innerHTML
1 |
|
2.2 显示切换,属性绑定
v-show
- v-show 指令的作用是根据表达式的真假,切换元素的显示和隐藏
- 修改元素的 display,实现显示隐藏
- 指令后面的内容,最终都会解析为布尔值
- 值为 true 元素显示,值为 false 元素隐藏
- 数据改变之后,对应元素的显示状态会同步更新
1 |
|
v-if
- v-if 指令的作用是:根据表达式的真假,切换元素的显示和隐藏(操作dom元素)
- 本质是通过操纵 dom 元素来切换显示状态
- 表达式的值为 true,元素存在于 dom 数中,为 false,从 dom 树中移除
- 频繁的切换 v-show,反之使用 v-if,前者切换消耗小
1 |
|
v-bind
- v-bind 指令的作用是:设置元素的属性(比如:src,title,class)
- 完整写法式 v-bind:属性名
- 简写的话可以直接省略 v-bind,只保留 :属性名
- 需要动态的增删 class 建议使用对象的方式
1 |
|
实例
- 列表数据使用数组保存
- v-bind 指令可以设置元素属性,比如 src
- v-show 和 v-if 都可以切换元素的显示状态,频繁切换用 v-show
1 |
|
2.3 列表循环,表单元素绑定
v-for
- v-for 指令的作用是:根据数据生成列表结构
- 数组经常和 v-for 结合使用
- 语法是 (item,index) in 数据
- item 和 index 可以结合其他指令一起使用
- 数组长度的更新会同步到页面上,是响应式的
1 |
|
v-on 补充
传递自定义参数,事件修饰符
文档传送门:https://cn.vuejs.org/v2/api/#v-on
- 事件绑定的方法写成函数调用的形式,可以传入自定义参数
- 定义方法时需要定义形参来接收传入的实参
- 事件的后面跟上 .修饰符 可以对事件进行限制
- .enter 可以显示触发的按键为回车
- 事件修饰符有多种
1 |
|
v-model
v-model 指令的作用是便捷获取和设置表单元素的值(双向数据绑定)
绑定的数据会和表单元素相关联
绑定的数据:left_right_arrow:表单元素的值
1 |
|
实例(记事本)
功能:
- 新增:
- 生成列表结构(v-for 数组)
- 获取用户输入(v-model)
- 回车,新增数据(v-on.enter 添加数据)
- 删除:
- 点击删除指定内容(v-on splice 索引)
- 统计:
- 统计信息个数(v-text length)
- 清空:
- 点击清除所有信息(v-on)
- 隐藏:
- 没有数据时,隐藏元素(v-show v-if 数组非空)
重点:
- 列表结构可以通过 v-for 指令结合数据生成
- v-on 结合事件修饰符可以对事件进行限制,比如 .enter
- v-on 在绑定事件时可以传递自定义参数
- 通过 v-model 可以快速的设置和获取表单元素的值
- 基于数据的开发方式
1 |
|
三、网络应用
Vue结合网络数据开发应用
3.1 axios
功能强大的网络请求库
1 | <script src="https://unpkg.com/axios/dist/axios.min,js"></script> |
- axios 必须先导入才可以使用
- 使用 get 或 post 方法即可发送对应的请求
- then 方法中的回调函数会在请求成功或失败时触发
- 通过回调函数的形式可以获取相应内容,或错误信息
1 |
|
3.2 axios+vue
axios 如何结合 vue 开发网络应用
- axios 回调函数中的 this 已经改变,无法访问到 data 中数据
- 把 this 保存起来,回调函数中直接使用保存的 this 即可
- 和本地应用的最大区别时改变了数据来源
1 |
|
实例(天气查询)
功能:
- 回车查询
- 按下回车(v-on.enter)
- 查询数据(axios 接口 v-model)
- 渲染数据(v-for 数组 that 或使用箭头函数)
- 应用的逻辑代码建议和页面分离,使用单独的 js 文件编写
- axios 回调函数中 this 指向改变了,需要额外保存一份
- 服务器返回的数据比较复杂时,获取的时候需要注意层级结构
- 点击查询
- 点击城市(v-on 自定义参数)
- 查询数据(this.方法())
- 渲染数据(this.方法())
- 自定义参数可以让代码的复用性更高
- methods 中定义的方法内部,可以通过 this 关键字点出其他的方法
1 |
|
1 | var app = new Vue({ |
四、综合应用(播放器)
4.1 歌曲搜索
- 按下回车(v-on.enter)
- 查询数据(axios 接口 v-model)
- 渲染数据(v-for 数组 that 或使用箭头函数)
- 服务器返回的数据比较复杂时,获取的时候需要注意层级结构
- 通过审查元素快速定位到需要操纵的元素
4.2 歌曲播放
- 点击播放(v-on 自定义参数)
- 歌曲地址获取(接口 歌曲id)
- 歌曲地址设置(v-bind)
- 歌曲id依赖歌曲搜索的结果,对于不用的数据也需要关注
4.3 歌曲封面
- 点击播放(增加逻辑)
- 歌曲封面获取(接口 歌曲id)
- 歌曲封面设置(v-bind)
- 在vue中通过 v-bind 操纵元素
- 本地无法获取的数据,基本都会有对应的接口
4.4 歌曲评论
- 点击播放(增加逻辑)
- 歌曲评论获取(接口 歌曲id)
- 歌曲评论渲染
- 在 vue中通过 v-for 生成列表
4.5 播放动画
- 监听音乐播放(v-on play)
- 监听音乐暂停(v-on pause)
- 操纵类名(v-bind 对象)
- audio 标签的 play 事件会在音频播放的时候触发
- audio 标签的 pause 事件会在音频暂停的时候触发
- 通过对象的方式设置类名,类名生效与否取决于后面值得真假
4.6 mv播放
- mv图标显示(v-if)
- mv地址获取(接口 mvid)
- 遮罩层(v-show v-on)
- mv地址设置(v-bind)
4.7 综合应用重点
- 不同接口需要得数据是不同的
- 页面结构复杂之后,通过审查元素的方式取快速定位相关元素
- 响应式的数据都需要在 data 中定义
1 |
|
1 | /* |
- Post title:vue入门
- Post author:John_Frod
- Create time:2021-02-12 22:09:26
- Post link:https://keep.xpoet.cn/2021/02/12/vue入门/
- Copyright Notice:All articles in this blog are licensed under BY-NC-SA unless stating additionally.